Guide to 5-Channel Flame Sensor Module
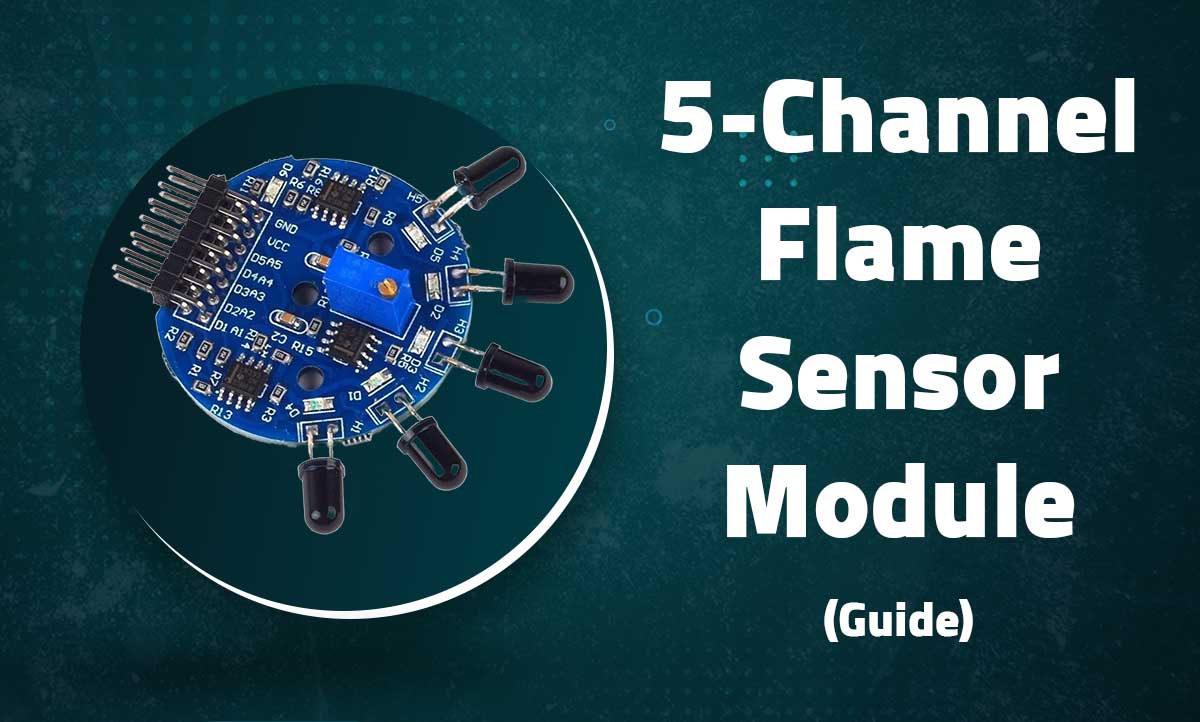
5-Channel Flame Sensor Module
Multi-directional Flame Detection for Arduino and Robotics Projects
Introduction
The 5-Channel Flame Sensor Module is an infrared detection system capable of identifying flame sources from multiple directions. With five independent IR receivers arranged in a 180° arc, it provides directional flame detection for fire prevention systems, robotics, and safety applications.
Key Features
Multi-directional
Five independent detection channels covering 180°
Analog Output
Provides intensity readings for precise flame location
Adjustable Sensitivity
Potentiometers for each channel’s detection threshold
Easy Integration
Works with 3.3V or 5V systems including Arduino
Technical Specifications
Detection Angle | 180° (5× 36° sectors) |
---|---|
Detection Range | 0.8m-1.5m (depends on flame size) |
Output Type | 5× Analog + 1× Digital |
Operating Voltage | 3.3V – 5V DC |
Current Consumption | <20mA per channel |
Response Time | <100ms |
Pin Configuration
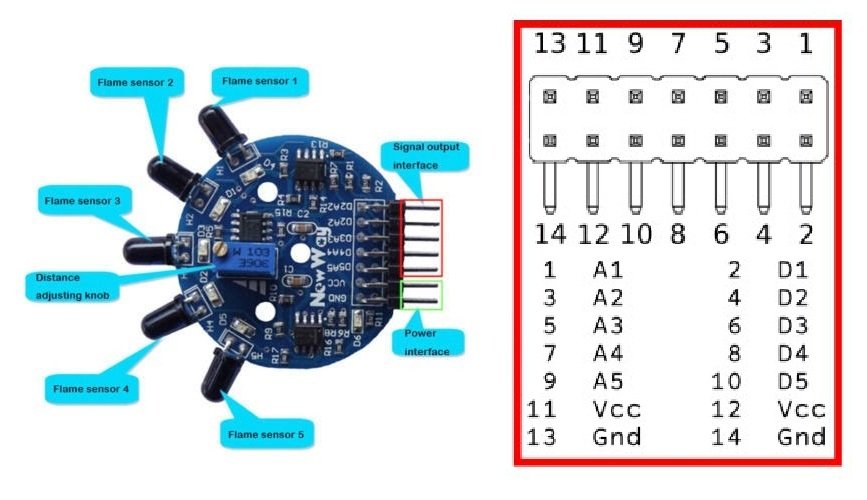
Pin | Label | Description | Arduino Connection |
---|---|---|---|
1 | VCC | Power (3.3V-5V) | 5V |
2 | GND | Ground | GND |
3 | A1 | Channel 1 Analog | A0 |
4 | A2 | Channel 2 Analog | A1 |
5 | A3 | Channel 3 Analog | A2 |
6 | A4 | Channel 4 Analog | A3 |
7 | A5 | Channel 5 Analog | A4 |
8 | D0 | Digital Output | D2 |
Note: Each channel has its own sensitivity adjustment potentiometer
Wiring with Arduino
1 2 3 4 5 6 7 |
// Basic Connections: // VCC → 5V // GND → GND // A1-A5 → A0-A4 (analog inputs) // D0 → D2 (digital input - optional) // For best results, place sensor 30-50cm above surface |
Important: Avoid direct sunlight as it may cause false positives
Basic Detection Example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// 5-Channel Flame Sensor Basic Example const int channels = 5; const int pins[channels] = {A0, A1, A2, A3, A4}; void setup() { Serial.begin(9600); for(int i=0; i<channels; i++) { pinMode(pins[i], INPUT); } } void loop() { for(int i=0; i<channels; i++) { int value = analogRead(pins[i]); Serial.print("Channel "); Serial.print(i+1); Serial.print(": "); Serial.print(value); Serial.print("\t"); } Serial.println(); delay(200); } |
Directional Flame Detection
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// Determine flame direction from 5 sensors int getFlameDirection() { int values[5]; int maxVal = 0; int direction = 0; // 0 = no flame // Read all channels for(int i=0; i<5; i++) { values[i] = analogRead(A0 + i); if(values[i] > maxVal && values[i] > 500) { // 500 = threshold maxVal = values[i]; direction = i + 1; // Channels 1-5 } } return direction; } void loop() { int flameDir = getFlameDirection(); if(flameDir > 0) { Serial.print("Flame detected from direction: "); Serial.println(flameDir); } delay(100); } |
Advanced Features
Threshold Calibration
1 2 3 4 5 6 7 8 9 10 |
// Auto-calibration for changing light conditions int baselines[5]; void calibrateSensors() { for(int i=0; i<5; i++) { baselines[i] = analogRead(A0 + i); // Add 10% safety margin baselines[i] += baselines[i] * 0.1; } } |
Flame Intensity
1 2 3 4 5 6 7 8 |
// Calculate relative flame intensity float getFlameIntensity() { int total = 0; for(int i=0; i<5; i++) { total += analogRead(A0 + i); } return total / 5.0; // Average intensity } |
Digital Output
1 2 3 4 5 6 7 8 9 10 |
// Using the digital output pin void setup() { pinMode(2, INPUT); // D0 connected to D2 } void loop() { if(digitalRead(2) { Serial.println("Flame detected!"); } } |
Robotic Response
1 2 3 4 5 6 7 8 9 10 11 |
// Simple robot fire-fighting logic void respondToFlame(int direction) { switch(direction) { case 1: turnLeft(45); break; case 2: turnLeft(15); break; case 3: moveForward(); break; case 4: turnRight(15); break; case 5: turnRight(45); break; } activateWaterPump(); } |
Troubleshooting
No Detection
- Adjust sensitivity potentiometers clockwise
- Check IR sensor alignment (should face outward)
- Verify flame is within 0.8-1.5m range
False Positives
- Reduce sensitivity (turn potentiometers CCW)
- Shield sensors from ambient IR sources
- Implement software debouncing in your code
Inconsistent Readings
- Ensure stable power supply (3.3V-5V)
- Check for loose wiring connections
- Recalibrate sensor baselines periodically