Guide to 4×4 Matrix Keypad for Arduino
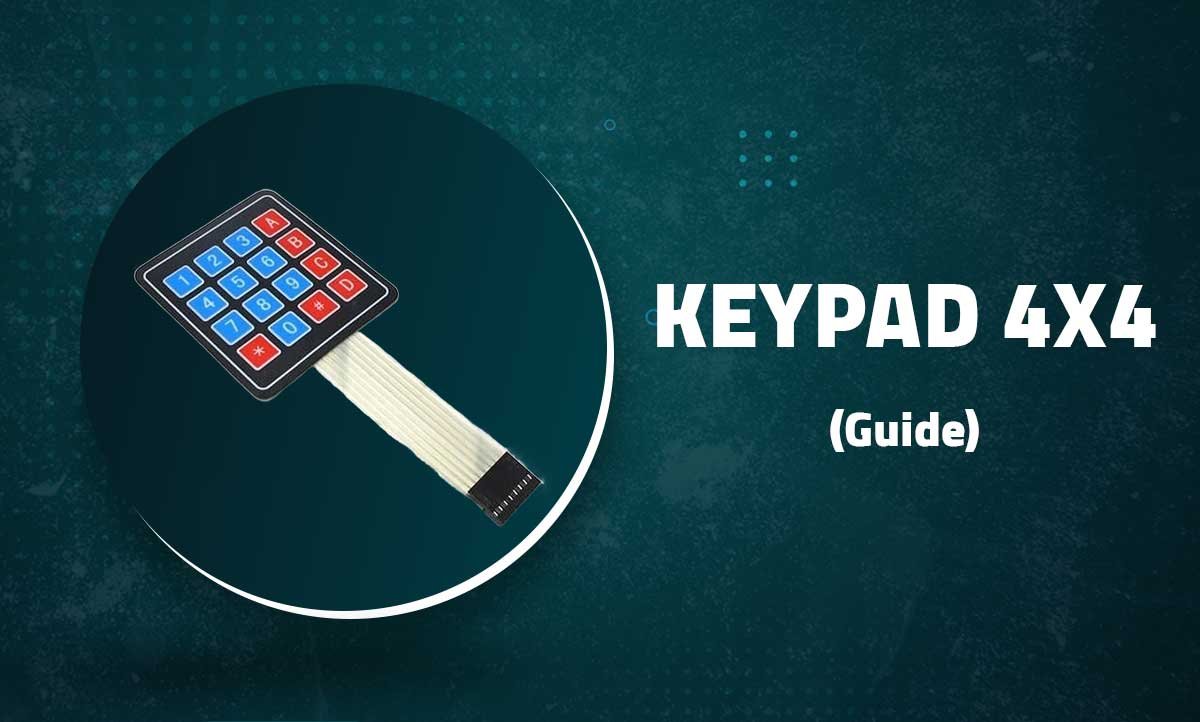
4×4 Matrix Keypad
16-Button Input Interface for Arduino and Microcontroller Projects
Introduction
The 4×4 matrix keypad is a compact input device with 16 buttons arranged in a grid. Using only 8 pins, it provides an efficient way to add multiple button inputs to Arduino, Raspberry Pi, and other microcontroller projects.
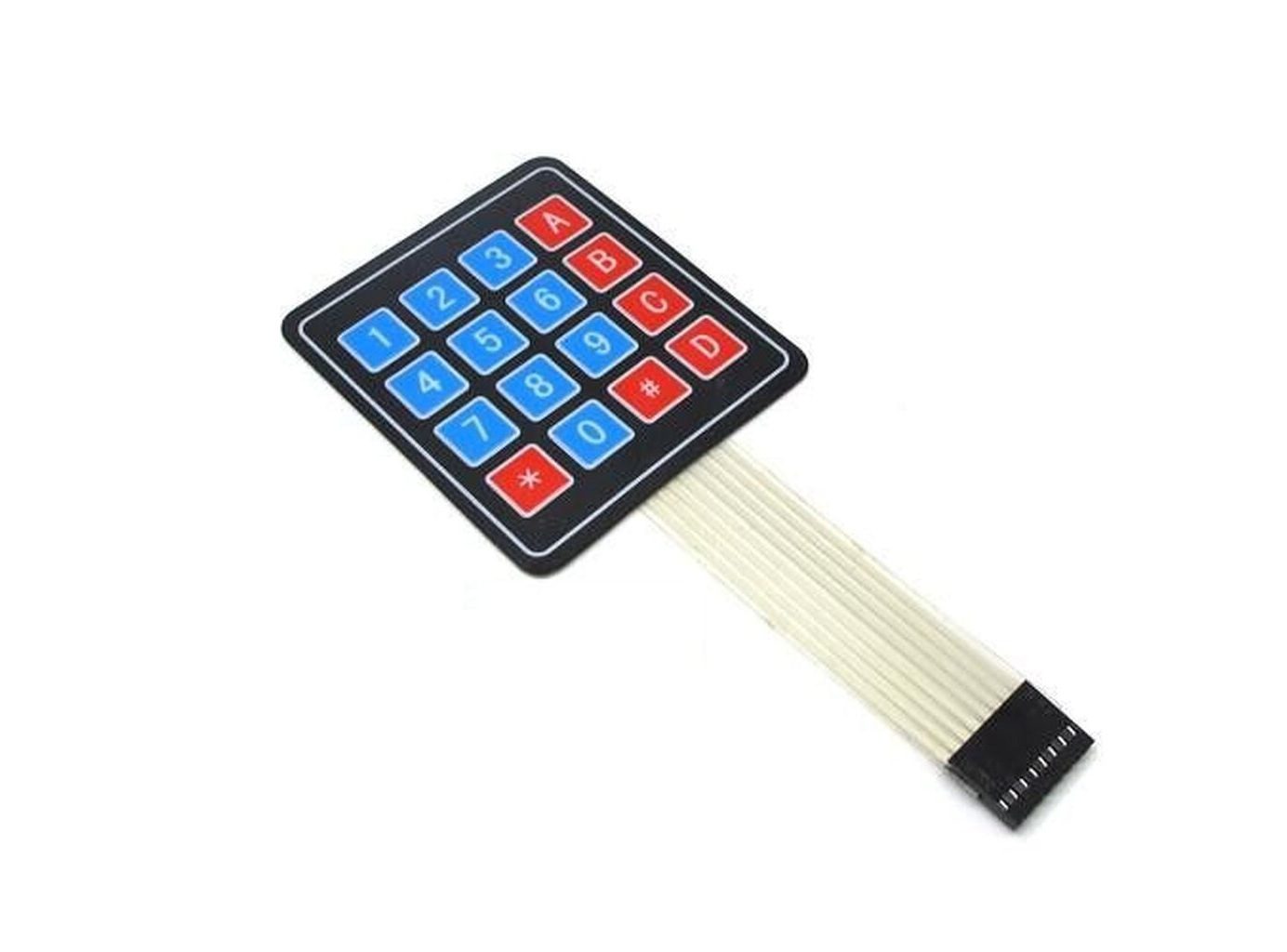
Key Features
16 Buttons
4 rows × 4 columns matrix configuration
Standard Layout
0-9 digits plus A,B,C,D,*,# symbols
Low Power
Only draws current during scanning
Simple Interface
8-pin connection (4 rows + 4 columns)
Technical Specifications
Operating Voltage | 1.5V – 24V DC |
---|---|
Contact Resistance | <100mΩ |
Insulation Resistance | >100MΩ |
Life Expectancy | 1,000,000 presses |
Dimensions | 80mm × 80mm × 10mm (typical) |
Button Spacing | 19mm × 19mm (standard) |
Pin Configuration
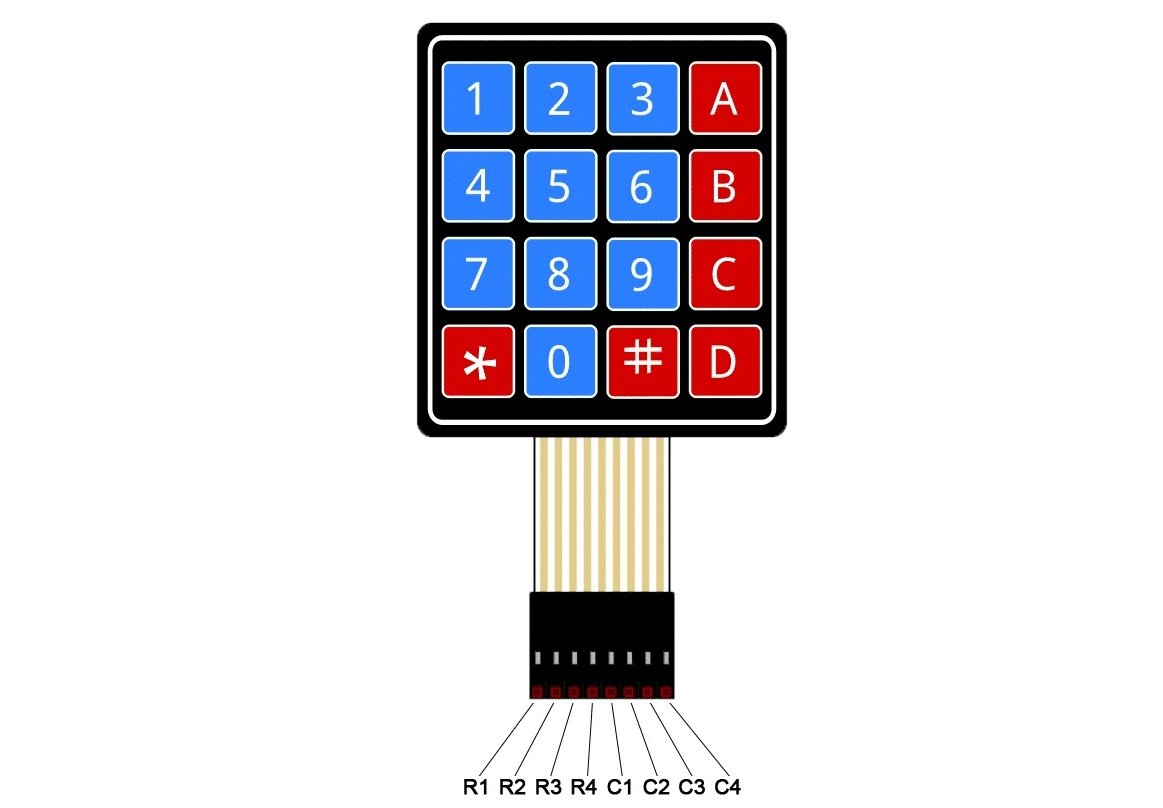
Pin | Function | Arduino Connection |
---|---|---|
1 | Row 1 | D9 |
2 | Row 2 | D8 |
3 | Row 3 | D7 |
4 | Row 4 | D6 |
5 | Column 1 | D5 |
6 | Column 2 | D4 |
7 | Column 3 | D3 |
8 | Column 4 | D2 |
Note: Pin connections can be customized to any digital pins
Wiring with Arduino
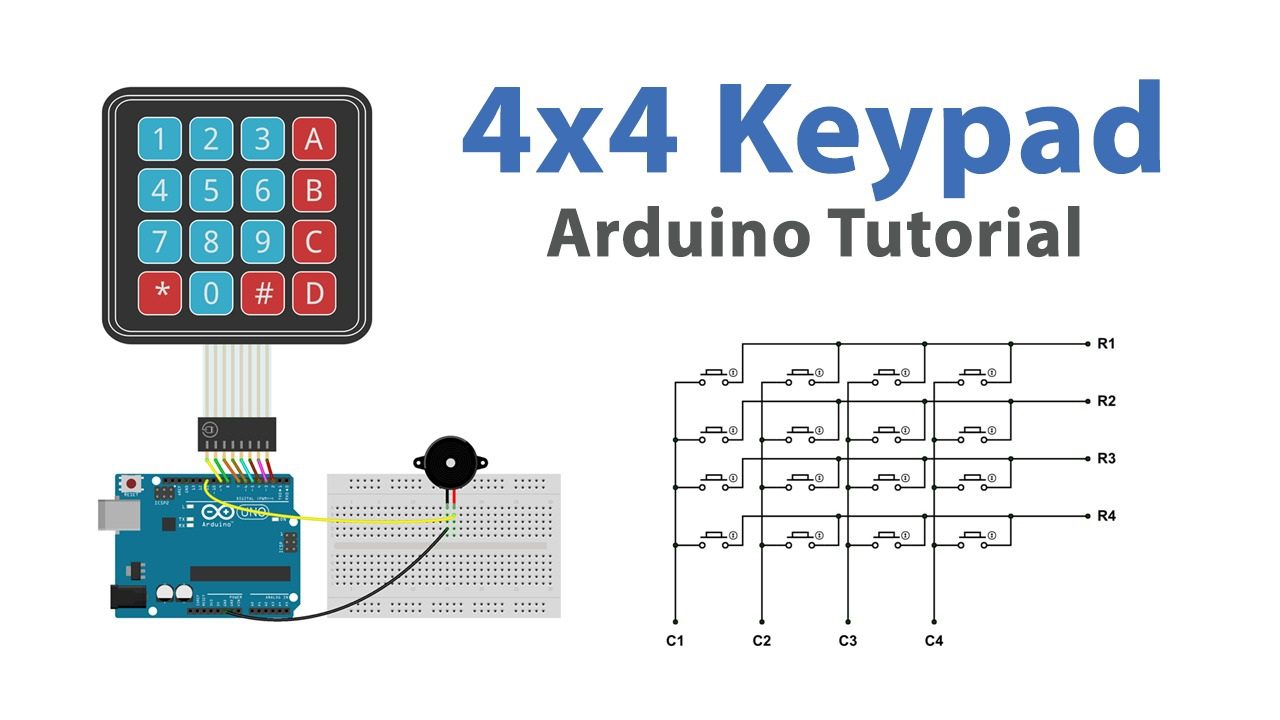
1 2 3 4 5 6 7 8 9 |
// Basic Connections: // Row 1 → D9 // Row 2 → D8 // Row 3 → D7 // Row 4 → D6 // Column 1 → D5 // Column 2 → D4 // Column 3 → D3 // Column 4 → D2 |
Important: Always use INPUT_PULLUP mode for columns to avoid floating inputs
Library Setup
- Install the Keypad library by Mark Stanley and Alexander Brevig
- Include the library in your sketch:
1#include <Keypad.h> - Configure the keypad layout:
12345678const byte ROWS = 4;const byte COLS = 4;char keys[ROWS][COLS] = {{'1','2','3','A'},{'4','5','6','B'},{'7','8','9','C'},{'*','0','#','D'}};
Basic Keypad Example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#include <Keypad.h> const byte ROWS = 4; const byte COLS = 4; char keys[ROWS][COLS] = { {'1','2','3','A'}, {'4','5','6','B'}, {'7','8','9','C'}, {'*','0','#','D'} }; byte rowPins[ROWS] = {9, 8, 7, 6}; byte colPins[COLS] = {5, 4, 3, 2}; Keypad keypad = Keypad(makeKeymap(keys), rowPins, colPins, ROWS, COLS); void setup() { Serial.begin(9600); } void loop() { char key = keypad.getKey(); if (key) { Serial.print("Pressed: "); Serial.println(key); } } |
Advanced Features
Password Entry
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
// Simple password check char password[4] = {'1','2','3','4'}; char entered[4]; int pos = 0; void checkPassword(char key) { entered[pos++] = key; if(pos == 4) { if(strncmp(entered, password, 4) == 0) { Serial.println("Access granted!"); } pos = 0; } } |
Keypad Events
1 2 3 4 5 6 7 8 9 10 11 |
// Detect key presses and releases void loop() { if(keypad.getState() == PRESSED) { Serial.print("Pressed: "); Serial.println(keypad.getKey()); } if(keypad.getState() == RELEASED) { Serial.print("Released: "); Serial.println(keypad.getKey()); } } |
Multi-key Press
1 2 3 4 5 6 |
// Detect multiple simultaneous presses void loop() { if(keypad.isPressed('A') && keypad.isPressed('B')) { Serial.println("A+B combo pressed!"); } } |
Custom Keymaps
1 2 3 4 5 6 7 |
// Special function keys char keys[ROWS][COLS] = { {'F','F','F','F'}, {'F','F','F','F'}, {'F','F','F','F'}, {'R','F','E','F'} }; // F=Forward, R=Reverse, E=Emergency Stop |
Troubleshooting
No Key Detection
- Verify all connections are secure
- Check row/column pin assignments
- Ensure proper keymap configuration
Ghost Presses
- Use INPUT_PULLUP for columns
- Add 0.1μF capacitors between rows/cols and GND
- Implement software debouncing
Multiple Keys Not Working
- Check for short circuits between pins
- Verify sufficient power supply
- Test with different digital pins