Blogs
HC-05 Bluetooth Module with Arduino Guide
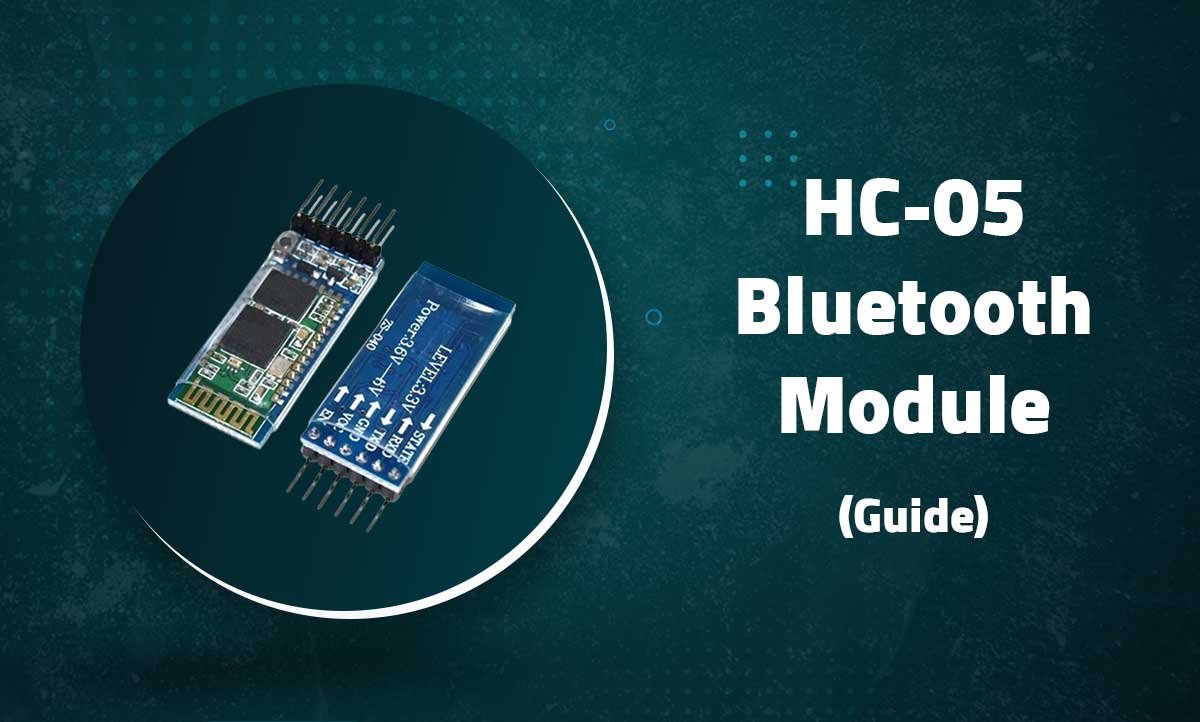
HC-05 Bluetooth Module Complete Guide
Wireless Communication for Arduino Projects
Introduction
The HC-05 Bluetooth module is a popular wireless communication device that allows microcontrollers like Arduino to communicate with smartphones, computers, and other Bluetooth-enabled devices. It operates on Bluetooth 2.0+EDR (Enhanced Data Rate) and supports serial communication (UART), making it easy to integrate into embedded projects.
HC-05 Module Description
Chipset: BC417 (Bluetooth v2.0)
Operating Voltage: 3.3V – 5V
Communication: UART at 9600 baud (default)
Range: Up to 10 meters
Modes: Master/Slave
Current: 30-40mA in operation
Key Features:
- Supports AT commands for configuration
- Works with Android & PC Bluetooth devices
- Low power consumption
- Easy serial communication
Applications
Wireless Sensor Data
Temperature, humidity, etc.
Bluetooth Robots
RC cars, robotic arms
Home Automation
Light control, fan speed
IoT Projects
Smartphone control
Connecting HC-05 with Arduino
Wiring Diagram
HC-05 Pin | Arduino Pin |
---|---|
VCC | 5V (or 3.3V) |
GND | GND |
TX | RX (Pin 0) |
RX | TX (Pin 1) |
⚠️ Important: When using 5V Arduino, use a voltage divider for RX line.
Arduino Code Example
#include <SoftwareSerial.h>
#define BT_TX 10
#define BT_RX 11
SoftwareSerial bluetooth(BT_TX, BT_RX);
void setup() {
Serial.begin(9600);
bluetooth.begin(9600);
Serial.println("Bluetooth Ready!");
}
void loop() {
if (bluetooth.available()) {
char received = bluetooth.read();
Serial.print("Received: ");
Serial.println(received);
}
if (Serial.available()) {
bluetooth.write(Serial.read());
}
}
AT Command Configuration
Basic AT Commands
Command | Response | Description |
---|---|---|
AT | OK | Test connection |
AT+NAME? | +NAME:HC-05 | Get device name |
AT+NAME=MyBT | OK | Change name |
AT+PSWD? | +PSWD:1234 | Get PIN |
Note: Baud rate for AT mode is typically 38400
Troubleshooting
❌ No response to AT commands
- Check EN/KEY pin is held high
- Try different baud rates
❌ Can't pair after changes
- Reset module (AT+RESET)
- Reboot Arduino